Vue Js Change Position of Elements of Array: In Vue.js, you can change the position of elements in an array using several array methods like splice()
, push()
, pop()
, shift()
, and unshift()
. The splice()
method can be used to add or remove elements from an array at a specific position, while push()
and pop()
add or remove elements from the end of an array. Similarly, shift()
and unshift()
add or remove elements from the beginning of an array. By using these methods with appropriate parameters, you can easily change the position of elements in an array in Vue.js.
How can the splice method in Vue.js be used to change the position of an item within an array?
This code uses Vue.js to create a simple web application. It defines an array of items and an empty array called “reorderedItems”. It also defines a method called “moveItem” that moves an item from one index in the “items” array to another index and updates the “reorderedItems” array with the new order. The method uses the JavaScript “slice” method to make a copy of the “items” array, and the “splice” method to move the item from index 3 to index 4. The new order is then assigned to the “reorderedItems” array.
Vue Js Change Position of Item of Array Example
<script type="module">
const app = new Vue({
el: "#app",
data: {
items: ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5'],
reorderedItems: [] // We'll populate this array with the reordered items
},
methods: {
moveItem: function () {
// Create a copy of the original array
var itemsCopy = this.items.slice();
// Move the element at index 2 to index 4
itemsCopy.splice(2, 0, itemsCopy.splice(3, 1)[0]);
// Update the reorderedItems array with the new order
this.reorderedItems = itemsCopy;
}
}
});
</script>
Output of Above example
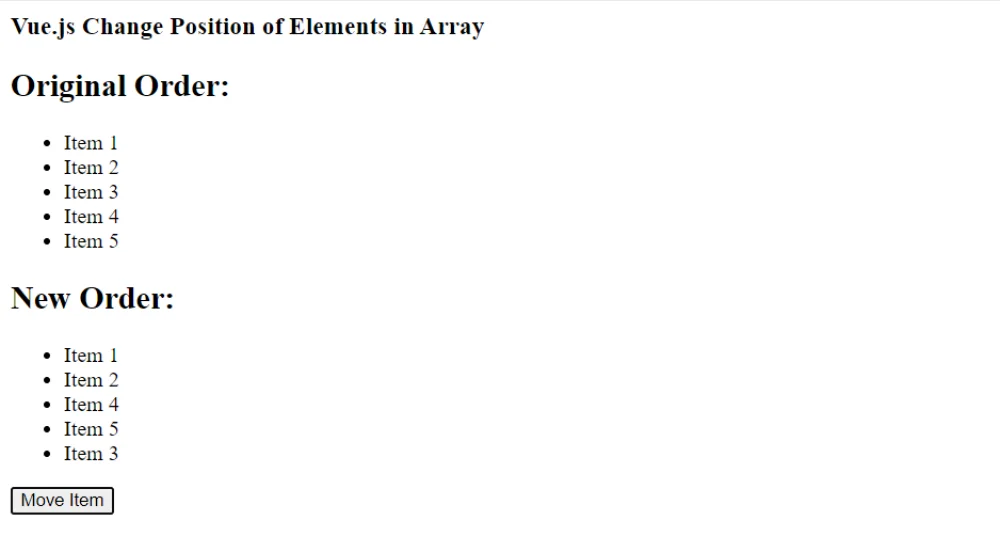